In software development, developers continually refine, enhance, and innovate. However, amidst this constant evolution, one essential practice often gets overlooked—code refactoring. Code refactoring is like the deep cleaning we do in our homes. We remove the clutter, organize our belongings, and make our living space more livable and efficient.
Like our homes, the codebase needs this level of maintenance to ensure it remains efficient, clean, and straightforward to navigate for any developer who might work on it.
What is code refactoring?
Code refactoring can be likened to cleaning up code without changing its functionality. It involves revising the existing code to make it more efficient and maintainable while ensuring that it doesn't alter the code's outward behavior. This process makes the code cleaner, more understandable, and easier to read, which in turn helps to locate and fix bugs faster.
Importance and Benefits of Code Refactoring
The benefits of code refactoring can be easily overlooked in the rush to deliver functionality or meet deadlines. Still, it is essential to a codebase's long-term health and maintainability. It's like regularly servicing a car: we may not see the immediate impact, but regular maintenance can prevent breakdowns and enhance performance.
Some of the key benefits include:
1. Improved code readability and reduced complexity
Code refactoring helps make the code more straightforward and, as a result, more understandable. This is crucial when new developers join the project or the original developers need to revisit their old code.
2. Easier bug detection
When code is clean and organized, it becomes much easier to spot anomalies or bugs that may have been hiding in the clutter. This reduces the time it takes to debug and resolve issues.
3. Enhanced code efficiency
Refactoring can help identify redundant code or areas where the code can be optimized for performance. This can lead to an overall increase in the application's speed and efficiency.
4. Reduced cost in the long run
While refactoring requires time and resources initially, it can lead to significant savings in the future. Cleaner and more efficient code means less time spent on debugging and more time adding new features.
To draw a parallel, imagine your codebase as a house. If things are clean and organized and every item is in its place, it's easier to move around, locate items, and keep the space functional.
The house analogy aligns perfectly with your codebase. A clean and organized codebase ensures developers can quickly identify and update codes, improve them, and fix bugs faster, keeping your 'code house' efficient and functional.
When Should You Consider Code Refactoring?
While refactoring should be a regular practice, specific scenarios call for it more urgently. Understanding when to refactor can make the process more effective and less disruptive.
Before adding any updates or new features
Refactoring before adding new features is like preparing your workspace before you start working. Just as a clean workspace increases productivity, a clean codebase makes it easier to implement new features.
In a study by Boehm and Basili, the cost of addressing complexity increases exponentially as the project progresses.
Therefore, refactoring before adding new features can significantly reduce costs in the long run.
Consider a software company that wanted to add a new feature to its application. However, the developers soon realized that the new feature's integration would require an overhaul due to the complex, entangled code.
The result was a deployment delay and increased costs. The team could have saved time and resources by practicing code refactoring before integrating the new feature.
Post-product delivery to the market
Refactoring can also be essential after delivering a product to the market. Once a product is live, you might identify performance issues, or the need for additional features might arise. Refactoring at this stage prepares the codebase for such enhancements.
For example, Twitter's shift from Ruby on Rails to JVM is a well-documented case of large-scale refactoring. The move was necessary to improve performance and scalability. It was a challenging and time-consuming process, but the refactoring paid off.
Today, Twitter serves hundreds of millions of users around the globe, demonstrating the importance of refactoring in scaling applications.
How to perform code refactoring: The main techniques
Choosing the correct method is an essential step in the refactoring process. Let's dive into some of the most popular techniques in the industry.
1. The Red-Green-Refactor method in Agile development
The Red-Green-Refactor method is a central tenet of test-driven development (TDD), a software development process that relies on repeating a concise development cycle.
This method can be visualized in three simple steps:
- Red: Write a test that fails.
- Green: Write minimal code to pass the test.
- Refactor: Improve the code while keeping the test green.
The book "Refactoring: Improving the Design of Existing Code" by Martin Fowler mentions a case study where a 250-person product team at Google used this method to refactor a significant portion of their codebase successfully. The developers could remove a substantial amount of outdated code and improve system performance, all without breaking the functionality.
2. Refactoring by abstraction: Pull-Up/Push-Down method
The Pull-Up and Push-Down methods help move methods between superclasses and subclasses when working with an inheritance hierarchy.
The pull-Up method moves a method from a subclass to its superclass, ensuring it is available to all subclasses. On the contrary, the Push-Down method moves a method from a superclass to a subclass if the technique is only relevant to that subclass.
A popular online payment platform reported improving its system efficiency by 25% using abstraction refactoring, enhancing its customer experience significantly.
3. Composing method: Extraction and inline methods
Composing methods involve breaking down more extensive, complex processes into smaller, more manageable ones.
Extraction Method
Here, we take a code fragment that can be grouped, move it into a separate method, and replace the old code with a call to the method. This makes the original process easier to understand.
For example, GitHub's Atom text editor extensively used extraction methods to enhance its maintainability and readability, reducing their bug count significantly over six months.
Inline Method
Conversely, Inline Method is a refactoring technique where the method's body replaces all calls to the method, and then we remove the method itself. It is an excellent technique to apply when the method body is as clear as the method name, or the method is overly trivial.
Inline method refactoring was employed in developing the Django web framework, simplifying complex codes, making them more readable, and reducing development time by up to 25%.
4. Simplifying methods
Simplifying methods is about making your code easier to read and understand. This could involve reducing the number of parameters a method requires, replacing parameters with explicit methods, or replacing error codes with exceptions.
Netflix's engineering team, for instance, used method simplification techniques in their API development to make their codebase more manageable and less error-prone, significantly reducing client-side errors.
5. Moving features between objects
Sometimes it might be beneficial to move a feature or method from one class to another to maintain each class's coherence or to balance the distribution of responsibilities among classes. This practice helped Spotify streamline its music recommendation algorithm, leading to a 30% improvement in recommendation relevancy.
6. Preparatory refactoring
Sometimes, developers must clean up the code before adding new features to make the change easy. This preparatory refactoring can involve any of the techniques discussed earlier.
When MailChimp was preparing to introduce a significant feature update, it undertook a two-week refactoring sprint using the preparatory refactoring technique. As a result, it successfully launched the feature without any essential issues, increasing its active user base by 18%.
For businesses seeking other options, exploring Mailchimp alternatives can also offer valuable solutions.
When you don’t need refactoring
Sometimes, your codebase may need to be updated or riddled with deep-rooted issues; a complete application rewrite might be a more effective solution.
This was the case for Slack, which undertook a substantial app rebuild for its desktop client in 2019 to overcome performance and maintainability issues.
In time-sensitive projects, the cost of refactoring may outweigh the benefits. For instance, if a healthcare tech company is under a tight deadline to deliver an app for tracking pandemic-related data, extensive refactoring could delay the app's critical launch.
Best practices for code refactoring
These proven strategies help ensure refactoring success and mitigate any potential risks involved in the process.
1. Refactor first before adding any new features
This practice ensures the codebase is in the best possible state to accommodate new additions. Instagram's development team followed this approach before introducing their IGTV feature, helping to prevent feature creep and maintain a clean, manageable codebase.
2. Planning your refactoring project and timeline carefully
This involves deciding what to refactor, when, and how to mitigate associated risks. Twitter, for example, carefully planned its shift from Ruby on Rails to a JVM-based stack over several years, allowing for a smooth transition without significant user-facing issues.
3. Testing often throughout the refactoring process
Testing is a crucial part of refactoring. Netflix has automated tests that run continuously throughout their refactoring process, enabling them to catch and address any regressions or issues immediately.
4. Involving your QA team in the process
The QA team plays a vital role in ensuring the refactored code meets the quality standards. Companies like Shopify involve their QA teams early to ensure thorough testing and high-quality code.
5. Focusing on progress, not perfection
Remember, refactoring is about improving the codebase incrementally, not perfecting it. Microsoft follows this principle, regularly making minor improvements to its vast codebase.
6. Trying refactoring automation
There are tools available that can automate parts of the refactoring process. Google, for instance, developed an internal tool called Rosie, which has automated millions of refactorings, improving developer productivity and code quality.
Best Practices for Code Refactoring (Bonus tips)
We've already discussed several best practices developers should follow when refactoring code. Here are a few more.
1. Take incremental steps
Rather than attempting to refactor a large portion of your codebase simultaneously, taking small, incremental steps is often best. This approach makes the process more manageable and reduces the risk of introducing bugs. Amazon applies this principle by frequently deploying small but substantive changes to its codebase, leading to continuous improvement and allowing them to maintain one of the world's most complex e-commerce platforms.
2. Keep your Code DRY (Don't Repeat Yourself)
Duplication is the root of all evil in programming. DRY is a principle of software development aimed at reducing repetition. Applying DRY principles means that developers should aim to reduce the repetition of information of all kinds. Twitter's development team follows this principle, which has helped to keep its codebase clean and maintainable despite its large size.
3. Use refactoring tools
Many integrated development environments (IDEs) include tools that automate refactoring tasks. This can speed up the refactoring process and help ensure you don't introduce bugs in your code. Airbnb, for example, uses the RuboCop tool for their Ruby codebase to enforce style conventions and find common errors before they become problems.
Moving forward
Code refactoring is vital to modern software development, enabling teams to maintain clean, efficient, and manageable codebases. By understanding when and how to refactor code effectively - and when not to - you can significantly enhance the quality of your software, reduce bugs, and make your code easier to understand and maintain.
Whether you're working on a small personal project or an extensive commercial application, following the best practices outlined in this article will help you get the most out of your refactoring efforts. And remember, refactoring is an ongoing process - there's always room for improvement.
Level up Your Codebase with Code Refactoring
Ready to take your codebase to the next level? Contact us at Rapidops and discover the power of code refactoring. Improve code readability, reduce complexity, and optimize efficiency for a more maintainable and cost-effective application. Let's refactor your code and unlock its full potential.
Frequently Asked Questions (FAQs)
Now that we've covered the main topics, let's address some of the most Frequently Asked Questions.
What is the best practice for refactoring code?
What is the best practice for refactoring code?What is the golden rule of three refactoring?
What is the golden rule of three refactoring?How should developers integrate refactoring into their workflow?
How should developers integrate refactoring into their workflow?What is the code refactoring process?
What is the code refactoring process?What is the first rule of refactoring?
What is the first rule of refactoring?What development practice helps developers integrate their code into the repository multiple times a day?
What development practice helps developers integrate their code into the repository multiple times a day?
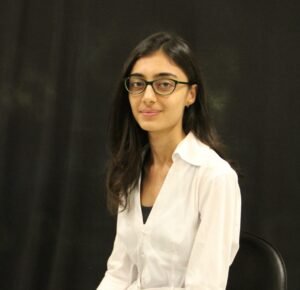
Niyati Madhvani
A flamboyant, hazel-eyed lady, Niyati loves learning new dynamics around marketing and sales. She specializes in building relationships with people through her conversational and writing skills. When she is not thinking about the next content campaign, you'll find her traveling and dwelling in books of any genre!
What’s Inside
- What is code refactoring?
- When Should You Consider Code Refactoring?
- How to perform code refactoring: The main techniques
- When you don’t need refactoring
- Best practices for code refactoring
- Best Practices for Code Refactoring (Bonus tips)
- Moving forward
- Level up Your Codebase with Code Refactoring
- Frequently Asked Questions (FAQs)
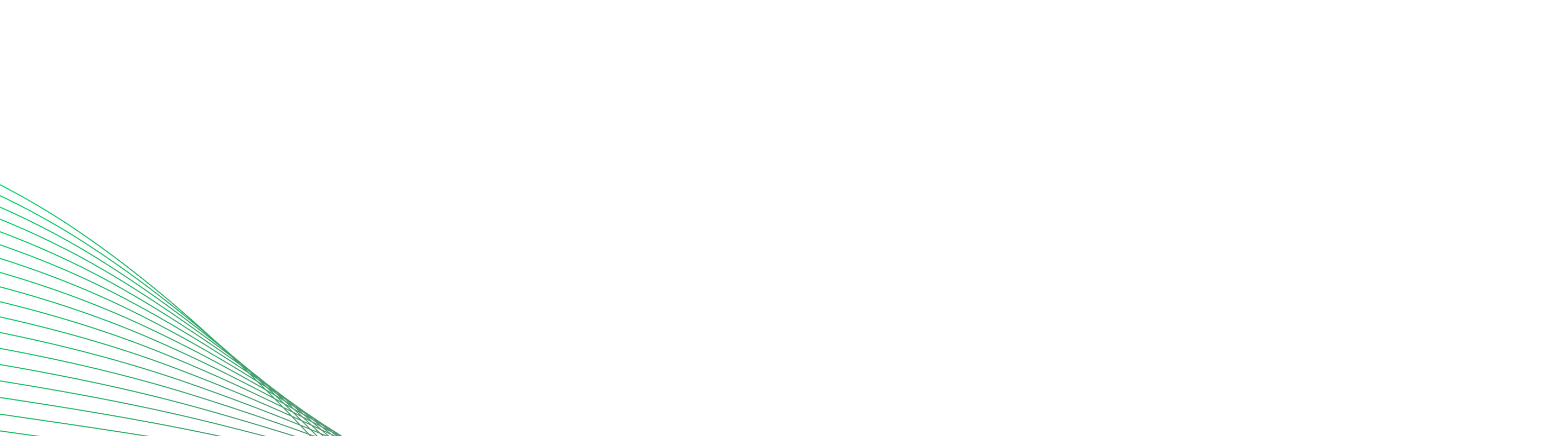
Let’s build the next big thing!
Share your ideas and vision with us to explore your digital opportunities
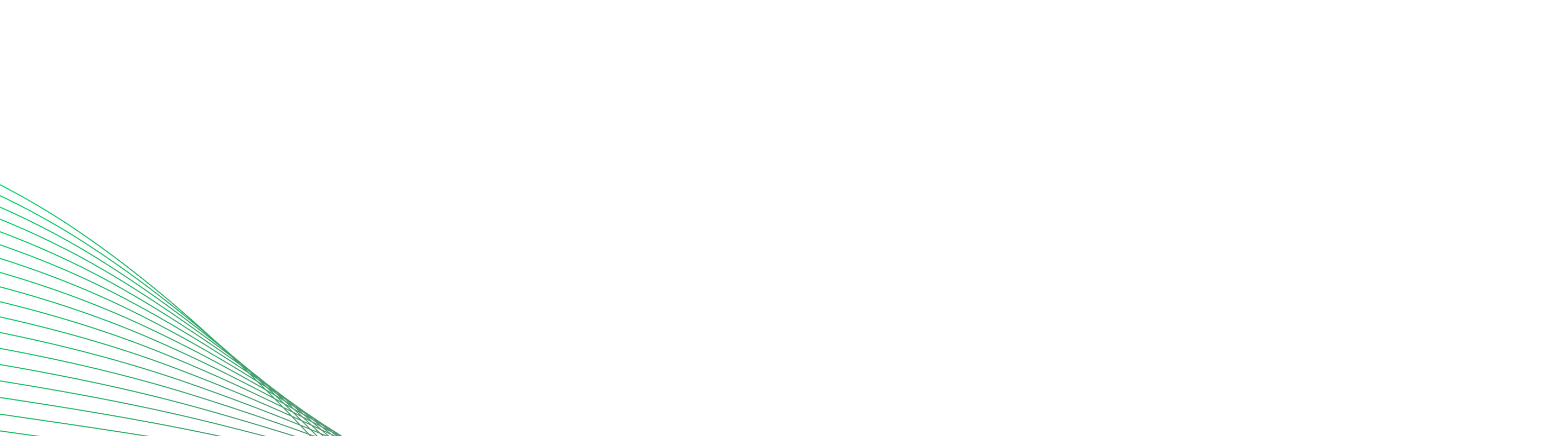
Receive articles like this in your mailbox
Sign up to get weekly insights & inspiration in your inbox.